Introduction to React without JSX
From a few previous versions of React JSX is not a requirement. Using React without JSX is convenient when we set up bigger projects. JSX is an Extension Syntax that allows writing HTML and Javascript together easily in React.
Take a look at the below code:
const start = <h1> Here it comes, JSX </h1>
Although this is a simple JSX in React, the browser does not recognize it since it is not genuine Javascript code. That's why an HTML tag is applied to a variable that isn't a string but rather HTML code. So we utilize a tool like Babel, a JavaScript compiler/transpiler, to turn it into browser-friendly JavaScript code.
Props and PropTypes are useful for passing read-only values between React components. Click to explore about, ReactJs Typechecking with PropTypes
What is JSX?
Although using JSX isn't required by React, most people find it useful as a visual aid when dealing with UI in JavaScript code. Using this React can now provide more informative error and warning messages. Consider this variable declaration example:
const tag = <h1>Bonjur! world!</h1>;
This tag syntax is neither an HTML nor a string, called JSX, extension to JavaScript.
Why do we use JSX in ReactJS?
Why JSX? Because React recognizes that rendering logic is inextricably linked to other UI logic, such as how events are handled, state changes over time, and data preparation for display.
React separates issues through loosely connected units called "components" that incorporate both, rather than artificially dividing technologies by putting markup and functionality in separate files.
React doesn't follow any particular project structure, and the positive thing about this is that it allows us to make up a structure to suit our needs. Click to explore about, ReactJs Project Structure and Folder Setups
What gets JSX to switch?
There are various factors as listed below:
Not limited to HTML Tags
Yes! That’s right, not limited to HTML tags!
Indeed, you'll generate a lot of elements depending on your developed components, just like in JSX!
import React from 'react.'
const nextComponent = props => {
return React.createElement(currentComponent, null, null)
}
We build another React element in this example using the myComponent functional component defined earlier in this post.
As a result, React.createElement works with both HTML elements and custom components - the custom components can be functional (as in this article), but they can also be class-based (stateful).
From React Element to DOM
Function React.createElement creates a single instance of a component.
If you provide a string, it will turn it into a DOM tag with that string as the value. We utilized the tags h1 and div, which are output to the DOM.
The characteristics we're sending to the tag or component are represented by the second empty object (you can also put null).
-
ReactDOM.render is the function that takes our rendered App component and places it in the DOM (in our example, the root element).
-
React.createElement is used with App as an argument to ReactDOM.render.To render out, we'll need an instance of App. The app is a component class, and we only need to render one instance of it.React.createElement accomplishes exactly that: it creates a class instance.
So, we've got these React elements that we made using React.createElement.But how does it rendered in the DOM?
Same vanilla JS has a createElement method, too:
document.createElement('div') // Here it creates.. <div>
React.createElement produces a JS object with all the necessary configurations to render it to the real DOM. And ReactDOM, another module from the React ecosystem, is used to render the page.
ReactDOM.render(React.createElement(nextComponent, null, null),
document.getElementById(Appelement)
This will turn the newly generated React element into a hook that we've provided in our HTML page, into which this script and its React dependency will be imported:
<body>
<div id="Appelement"></div>
<script crossorigin src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="App.js"></script>
</body>
Setup requires no workflow build since having import and use vanilla JS only.
The splitting of code into components or numerous bundles which can be loaded when there is a demand or in parallel. Click to explore about, Code-Splitting in ReactJs
What about props?
When we create elements with React, createElement, the second argument was always null.
It looks like this:
React.createElement('div', { className: 'first-css-class' }, 'First ele')
This would lead HTML element being created:
<div class="first-css-class">An Element</div>
Generally, the second argument allows binding properties or passing props to the element we are creating. So the JSX equivalent would be this:
<div className="first-css-class">An Element</div>
Because class is a reserved keyword in JS, className is essential.
Also, we can pass our props to our components. Below mentioned a JSX example passed by the less-JSX alternative:
<Tag name="Jhon" age={31} />
React.createElement(Tag, { name: 'Jhon', age: 31 }, null)
Hello world without JSX
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Bonjur! World</title>
<script src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
</head>
<body>
<div id="root"></div>
<script type="text/javascript">
var e = React.createElement;
ReactDOM.render(
e('h1', null, 'Bonjur! world!'),
document.getElementById('root')
);
</script>
</body>
</html>
The second set of properties can be sent into that element (usually referred to as props). The third category is children's elements. In this example, we'll only type "Hello, world!" but we might easily add another element or component or, better yet, a list of them. Please copy and paste this into an HTML file & open it in your browser.
JSX is syntax so that you can write <h1>Hello, world!</h1> instead of React.createElement('h1', null, 'Hello, world!').
NodeJs is an open-source, server-side runtime atmosphere established on Google Chrome's V8 JavaScript transformer. Click to explore about, Node.js vs. Golang
Difference between React vs. Plain JavaScript?
The primary difference between plain JS and React apps is how each reacts to user interaction—for example, tapping a button to add a new item to a list—and then adjusting the UI accordingly.
Next to the input field, we could add a button in a standard JS app with the id:
<input type="text" placeholder="Enter any item" id="item" /> <button id="button">Add</button>
To respond to a button press, we must first locate the button in the DOM (like we did previously with input):
const add = document.getElementById("button");
And then, On that button, set a click listener:
addTap.addListener("click", function() {
//opt. Append here
})
Then, using the same way as before, inside click listener, we could access the value of the input box. In the grocery list for adding a new item, we must first locate it in the DOM, create the new item to be added, and append it to the end.
(Using some libraries, you can make this a little easier to do - but here is how you can do it with simply JavaScript code)
addButton.addEventListener("click", function () {
const input = document.getElementById("input");
console.log(input.value);
const list = document.getElementById("lugguage-list");
const longNode = document.createElement("li");
const inputNode = document.createTextNode(input.value);
listNode.appendChild(inputNode);
list.appendChild(longNode);
});
addButton.addEventListener("click", function () {
const input = document.getElementById("input");
console.log(input.value);
const list = document.getElementById("lugguage-list");
Const NodeList = document.createElement(‘li’);
const Nodetext = document.createTextNode(input.value);
Nodelist.appendChild(inputNode);
list.appendChild(longNode);
});
In contrast, a React app will be configured to store the whole state of the list in a JS variable.:
const [list, setList] = useState(["apple", "orange", "toasts"]);
Which will then be displayed in JSX by mapping (looping) over each item and returning a list element for each one:
<ul>
{list.map(item => (
<li key={item}>{item}</li>
))}
</ul>
<ul> {List.map(item => ( <li key={item}>{item}</li> ))} </ul>
The actual button press can then be specified directly in the code function. That implies you don't need a click listener, but you may add a onClick attribute to the button itself:
<button onClick={addList}>Add Your React Project</button>
And all that function has to do is use the setItems updater function to append the new item (stored in JavaScript memory) to the existing array of items.
function addItem() {
setItems([...List, listInput]);
console.log(listInput);
}
React will automatically detect that the list has changed and update the UI accordingly.
Because React apps are self-updating, you won't have to go into the DOM to figure out where to attach your items—it will be done for you automatically.
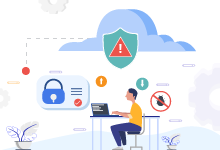
Conclusion
Using ReactJS without JSX can help you better grasp what Babel is doing behind the scenes. However, JSX will provide more simplicity and ease while doing a size project. The obvious question is whether you should utilize this JSX-less method instead of the JSX-focused approach seen in most React applications.
For medium-sized or larger projects, you should usually stick to JSX.
It's still useful to know what's going on underneath the hood. You might use the JSX-less version for smaller React projects or multi-page applications when you don't want to create a complex build sequence. It rapidly gets you up and running, and all you need are two React imports (React and ReactDOM) and your script file to get started. That doesn't sound like the worst option.
- Explore here about ReactJs Testing of Components
- Discover more about React.JS Application Development Services