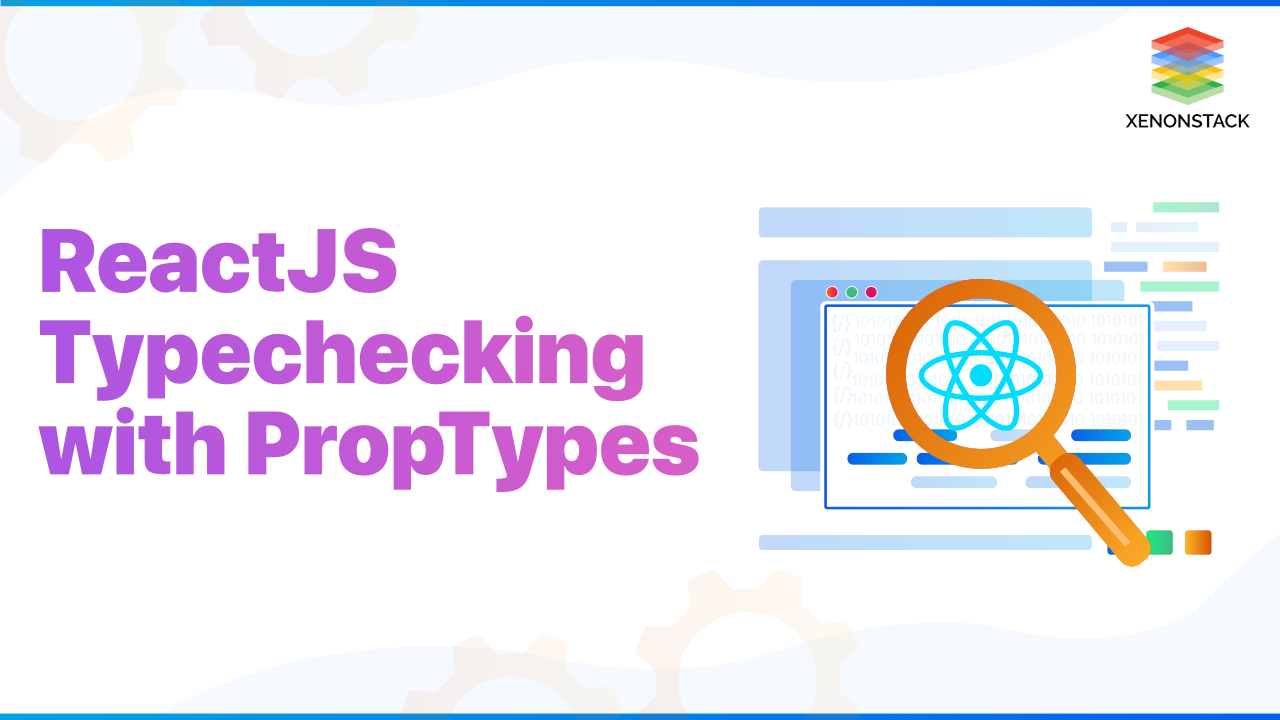
What is TypeChecking?
Types are one of a programming language's most important foundations. Errors in defining them can completely wreck a programme. Many JavaScript execution failures are type mistakes, such as dividing by a string.
With typechecking, you can catch many issues as your app expands. You can use JavaScript extensions like Flow or TypeScript to typecheck your entire programme in some cases. React comes with certain built-in typechecking features even if you don't use them. You can use the propTypes property to do typechecking on a component's props:
Because JavaScript lacks a built-in type-checking solution, many programmers rely on extensions like TypeScript and Flow. On the other hand, React offers a built-in mechanism for validating props called PropTypes.
CSS font size adjusts all the measurement units in which font sizes are present, like rem, em, px and viewport units. Click to explore about, CSS Size Adjust for Font Face
What is PropTypes?
Props and PropTypes are useful for passing read-only values between React components. Data is sent from one component using React props, which stands for "properties." If a component receives the incorrect props, your app may have issues and unexpected errors.
PropTypes are a mechanism for ensuring that components use the correct data type and pass the appropriate data, as well as that components, use the appropriate prop type and that receiving components receive the appropriate prop type.
A warning will appear in the JavaScript console if an invalid value is provided for a prop. propTypes is only tested in development mode for performance reasons.
Why use React PropTypes?
- PropTypes does type verification in the browser while the application is executing. When TypeScript code is compiled to JavaScript, TypeScript does type-checking at compile time. This is significant because it impacts how the tools can be used.
- PropTypes' primary benefit is that it's shared with other type checkers. It makes it simple to detect issues caused by data passing in the incorrect data type (like a string in a Boolean space).
- PropTypes has an advantage because it is already included in React. It's ready to use and has a low learning curve. Anyone who uses the component in the future will find all accessible props in one location, including their selected data type.
- Because certain code editors provide code completion for props, you can see the available props in a tooltip while typing in the component.
- PropTypes allow you to validate your props, which would otherwise be impossible due to JavaScript's loose typing.
- Props can be used anywhere they are needed, including by parents. Alternatively, I don't have them at all. Props are frequently the sole means for parents to pass on values or ideas to their children and grandchildren (in fact, it is recommended).
The concept of components is central to React. A web page should be divided into components, with each component interacting with the others via props.
Typography is the color, contrast, alignment, size, and font that directly impact a web page's performance. Click to explore about, Fluid Typography Best Practices
How PropTypes Works or How we use PropTypes?
The built-in type-checking tool in React is called PropTypes. PropTypes are used both singular and plural because they are both the tool's name and reference to actual PropTypes.
Said, the tool determines the type of prop (string, number, undefined, null, boolean, or symbol). It then exports a set of validators that may be used to ensure that the information received is accurate.
- PropTypes was provided in the react package before React 15.5.0. However, later versions of React need you to add a dependency to your project.
Using the command below, you can add the dependency to your project:
npm install prop-types --save
- The propType can validate any data received from props. However, before we can use it, we must first import it.
import PropTypes from 'prop-types'; - We're ready to work with propTypes now that we've imported them. PropTypes, like defaultProps, are objects with keys that are prop names and values that are prop types.
The following syntax demonstrates how to utilise propTypes:
The ComponentClassName in the above Syntax is the name of the Component class, and anyOtherType can be any type that we are allowed to supply as props.
Default Props
React has a feature called defaultProps that allows us to send some default information to our components via props. We can set defaultProps in circumstances where PropTypes are optional (i.e., they don't use isRequired). If no value is given, default props ensure that props have a value. Here's an illustration:
Suppose the parent component does not specify this.props.name, defaultProps will be used to ensure that it has a value. If the class Profile is not given a name, it will fall back on the default property Stranger. When no prop is supplied, this prevents any errors. For every optional PropType, I recommend using default props.
The FOIT is the loading mechanism that decides the text area is to be kept blank until the custom fonts are loaded. Click to explore about, Optimizing Layout Shifting and Flashes of Invisible Text
Why do we validate props in ReactJs?
When creating a React application, you may find that a prop needs to be structured and described to avoid faults and failures. A React component may require a prop to be defined, similar to how a function may have obligatory arguments; otherwise, it would not render correctly. If you fail to send a needed prop to a component that requires it, your app can act strangely.
For example, the component named PercentageStat in the above snippet requires three props for appropriate rendering: label, score, and total. If no values are provided for the score, and total props, default values are used. In the App component, PercentageStat is rendered four times, each with a different set of props.
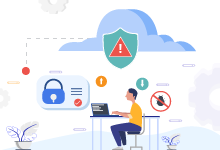
Conclusion
Hopefully, it has demonstrated the importance of props and propTypes in the development of React apps because, without them, we wouldn't be able to transmit data between components during interactions. They are an essential component of React's component-driven and state-management architecture.
PropTypes benefit from ensuring that components use the correct data type and pass the appropriate data. That component uses the appropriate prop type and receives the appropriate prop type.
- Discover more about API Testing Tools and Best Practices
- Discover more about Customer Experience and User Experience