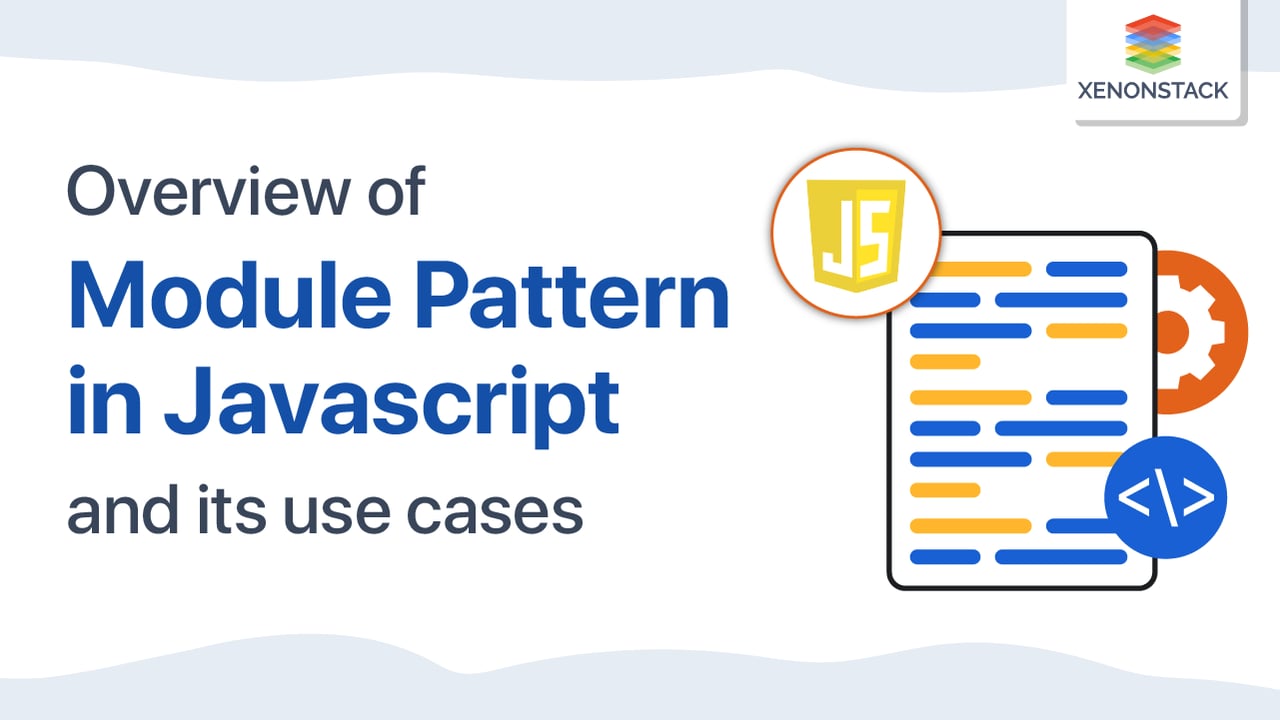
Introduction to Module pattern in Javascript
It is a functional programming language, which means that we can write code in Javascript by following both the procedural-oriented paradigm and using the object-oriented paradigm.
Also, it can be used both as a client-side and server-side scripting language. So as we have seen, Javascript is one of the most powerful programming languages, so it is necessary to write maintainable and scalable code in it. To achieve this scalability, we use several design patterns, and here we will explore one design pattern called module pattern.
A programming paradigm in which programs are constructed entirely of pure functions, with no shared mutable state or side effects. Click to explore about, Functional Programming in JavaScript
What is Module Pattern?
It is a special Design pattern in which we use IFFI (Immediately invoked function expression), and we return an object. Inside of that object, we can have functions as well as variables.
And that functions can be used further with the Html elements, and with this, we can achieve encapsulation where we can create an abstraction between our real functions and the functions used with Html elements.
Why do we use it?
We can write a piece of code in various ways in Javascript. But what if we have to fix some bugs or change some code functionality after the code is written. If a developer has written a piece of code and that code is not scalable, he will be confused after some time about what is going on with this piece of code.
Or in general, we can say that different developers have different minds and approaches to writing code. Hence, it is very difficult for different developers to read others' code, understand it, and then make changes. So to fill this gap, some design patterns are introduced. One of them is the Module pattern.
Here written some pieces of code in it:-
let marksOfStudent=20;
function increaseMarks(){
return marksOfStudent++;
}
Although we can use this piece of code to increase students' marks anywhere in our file, here the scalability is not there if I try to find this code on my script or say after some time I would like to change some functionality in this so it would be a challenging task. So to overcome this problem, we will use the Module pattern.
An open-source, server-side runtime atmosphere established on Google Chrome's V8 JavaScript transformer. Click to explore about, Node.js vs. Golang
What are the various methods of Module Pattern?
The below described are its various methods:
Object Literal Notation
An object is an array of name/value pairs enclosed in parentheses. An object name can be a string or an identifier followed by a colon. Do not use commas after label/value pairs in objects. This may cause errors.
Using object literals helps to encapsulate and organize code. If you want to learn more about object characters, Rebecca Murphy has written extensively. In other words, if you choose this technology, you might be interested in a modular model. It still uses the object symbol but only the return value of the range function.
The Module Pattern
The modular model was initially designed to encapsulate the common software engineering process. Template modules create objects containing public and private methods and variables. This isolates parts of the object from the global scope, reducing the chance that the function name will conflict with other functions on the page.
The module template includes organizations that use "privacy" states and closures. It includes a mix of public and private methods and variables, protects parts from leaks globally, and provides a way to prevent accidental conflicts with other developer interfaces. This template will only return the public API and keep the rest private in the private API.
This provides a clean solution that protects the logic that does the heavy lifting, exposing only the interfaces that other parts of the application want to use. A pattern is very similar to an immediate function expression[1], except that it returns an object instead of a function.
It's worth noting that JavaScript has no real sense of "privacy" because it has no access modifiers, unlike some traditional languages. Technically, variables cannot be declared public or private, so we use function scope to simulate this concept. Variables or methods declared in a module template are only available within the module itself because of closures. However, a variable or method defined on a duplicate object is public.
AMD Modules
The most common implementation of this standard is RequestJS. Characteristic:
The syntax is a bit more complex, allowing AMD to work without the eval() (or compile step). For asynchronous loading
Main use: Browser
CommonJS Modules
This default implementation dominates in Node.js (Node.js modules have some functionality outside of CommonJS). Characteristic:
- Shortened phrase
- For synchronous charging
- Main use: Server
ECMAScript Harmony Modules
- The goal of the ECMAScript 6 module is to create a format that will satisfy both CommonJS and AMD users.
- Like CommonJS, it has a concise syntax, prefers a single export, and supports circular dependencies. Like AMD, it supports direct loading and asynchronous loading of configurable modules.
- Language integration allows ES6 modules to outperform CommonJS and AMD (more on that later).
- Their syntax is more concise than CommonJS. Their structures can be statically analyzed (for static inspection, optimization, etc.).
- Circular dependency support is better than CommonJS. The ES6 modularity standard consists of two parts. Declarative syntax (for import and export)
Programmatic Loader API: To configure module loading and conditional module loading.
The best practices that a programmer must follow to amplify code reusability in a framework. Click to explore about our, Design Patterns in Automation Testing
What are the other patterns we have in Javascript?
All the patterns we have in Javascript are listed below:
Constructor Pattern
Since we know that constructor is a function that initializes whenever we make an object, in it. There is three ways to make an object.
var object = {};
var object = Object.create(Object.prototype);
var object = new Object();
Singleton Pattern
The singleton pattern is used when we need only one class instance. For example, if we need an object in which we need a particular configuration. So, we do not need to create a new object in this case. Instead, we can use a singleton pattern.
Observer Pattern
Let us consider that we have a system where we have disparate parts. So to maintain this type of system, we have a pattern called the observer pattern. This pattern is used when we have an extensive application. To maintain great applications where we also use libraries like Angular js. This type of pattern is used. We have two significant parts in the observer design pattern: observer and subject.
Mediator Pattern
When we have a scenario where we have different parts of the application communicating, we can use the Mediator pattern. When making great applications where we have to manage a large amount of data through the dashboard, we can use this Mediator pattern.
Prototype Pattern
We know that javascript does not support the concept of inheritance through classes. Here we can use inheritance between objects using prototype-based programming using prototype patterns.
Command Pattern
If we have a scenario where we have to use many api service calls and let suppose we have to Change that api service so there will be a case wherever we have implemented that calls. There we have to change everything.
So here, we need an abstraction layer of an object which can differentiate two objects, one which calls that api and one which knows when to call that api service.
Facade Pattern
A facade pattern is used to create an abstraction layer between what is shown publicly and what is behind the curtains. This is something similar to it.
Functional programming is a significant paradigm shift in the software world over the past 10 years. Click to explore about, Reducing Maintenance Costs With Functional Programming
What are the advantages and disadvantages?
The below highlighted are the advantages and disadvantages of module pattern
Advantages
It is a good choice because it helps to modularize the code base, making it easier to maintain and extend. For developers with object-oriented backgrounds, stuffing code with objects is much more precise than true encapsulation, at least from a JavaScript perspective.
Second, the module pattern allows the public part of the code to access the private part of the class and prevents outsiders from accessing it.
Disadvantages
The model is great, but it has some flaws. First, it doesn't use memory as efficiently as the prototype models we see. Each function is loaded into memory only once when you use the prototype model. This is not the case with the modular model. Whenever a module creates a new object, a new copy of all functions of that module is loaded into memory. If you have a module with 10 functions and create 10 new instances of this object, now 100 functions are loaded into memory. To be fair, JavaScript runtime optimizations should alleviate performance issues, but it's worth noting that this is an essential difference between prototypes and modules.
A second limitation of the model is that the extensibility and replacement capabilities of the module are lost because the prototype model is no longer used. Because some members are private and anonymous, some developers find editing increasingly difficult.
Comparison between Module patterns with others?
We have a specific pattern where we have a function that calls itself whenever the page loads. And that function returns an object, and it is a type of abstraction of properties and methods inside that function. We can use this method to bring encapsulation into our code. If we see other design patterns there, no such abstraction concept is used, or this type of pattern is performed. So in this way, it is different from other patterns.
There can be several differences between module patterns and others.
- It uses the concept of IFFI (Immediately invoked function expression), whereas others do not use the concept.
- Abstraction and Encapsulation of object-oriented paradigms are taken into high consideration, whereas in others, the pattern is different. As here, we are not using the function and variable directly outside the blog, but we are using them through another function.
- One main difference between IFFI and other patterns is that IFFI is very easy to understand and implement compared to other design patterns.
- We can achieve more scalability and readability by following this Module pattern concept.
The Dependency Injection increases flexibility and modularity in the applications. Using it in the application makes the code flexible, testable, and mutable. Click to explore about, Angular Dependency Injection
Use Case of Module Pattern
Before discussing the use case of it, let us discuss some prerequisites of the module pattern. They are:-
IFFI (Immediately invoked function expression)
IFFI is a function that automatically invokes whenever our script executes. It is a very effective way or alternative to using a window.onload function.
<script>
(()=>{
//here we can write code which will automatically run as script load
})();
</script>
Object Literals
The second prerequisite to the module pattern is object literals. Object literals is the main part of oops through which we can implement and use the real world. The entity in the programming world. For example, we have a car with some color, size, price, name, etc. So with the help of object literal, we can express this real-world entity into a virtual one in our program. Let us see how this is implemented in our program.<script>
const car={
name:’farari’,
color:'red',
price:10000000,
length:'10m'
}
</script>
Use Case of Module Pattern
So now, as we have seen some of the prerequisites for it, let us see how to implement it?
Let us consider an Html code where we have to achieve a functionality where we click a paragraph and color change on hover through Javascript. Now here we want to achieve color functionality in paragraphs, on hover. So we will achieve it with javascript using it.<html>
<head>
</head>
<body>
<p id="para" onclick="changeToRed()">This is a paragraph.</p>
</body>
</html>
<script>
const colorChanged=(()=>{
function colorChange(){
const para=document.getElementById('para');
para.style.color="red";
}
return {
colorIsChanged:function(){
colorChange();
}
}})();
changeToRed=()=>{
colorChanged.colorIsChanged();
}
</script>
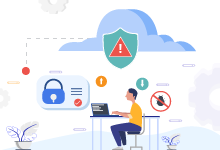
Conclusion
We can use any design pattern whatever we want, but if we are considering data abstraction and encapsulation paradigm, we should use the Module Design pattern. Module Design pattern uses IFFI (Immediately invoked function expression) to create a data abstraction layer. It only allows those properties and methods to use which are returned by the IFFI function expression. Hence data becomes more secure in the front end. Also, asymmetry in code is created to manage the code more efficiently if other developers are working on the same piece of code.
- Discover more about Functional Programming in Scala
- Read here about Functional Testing and Its Types