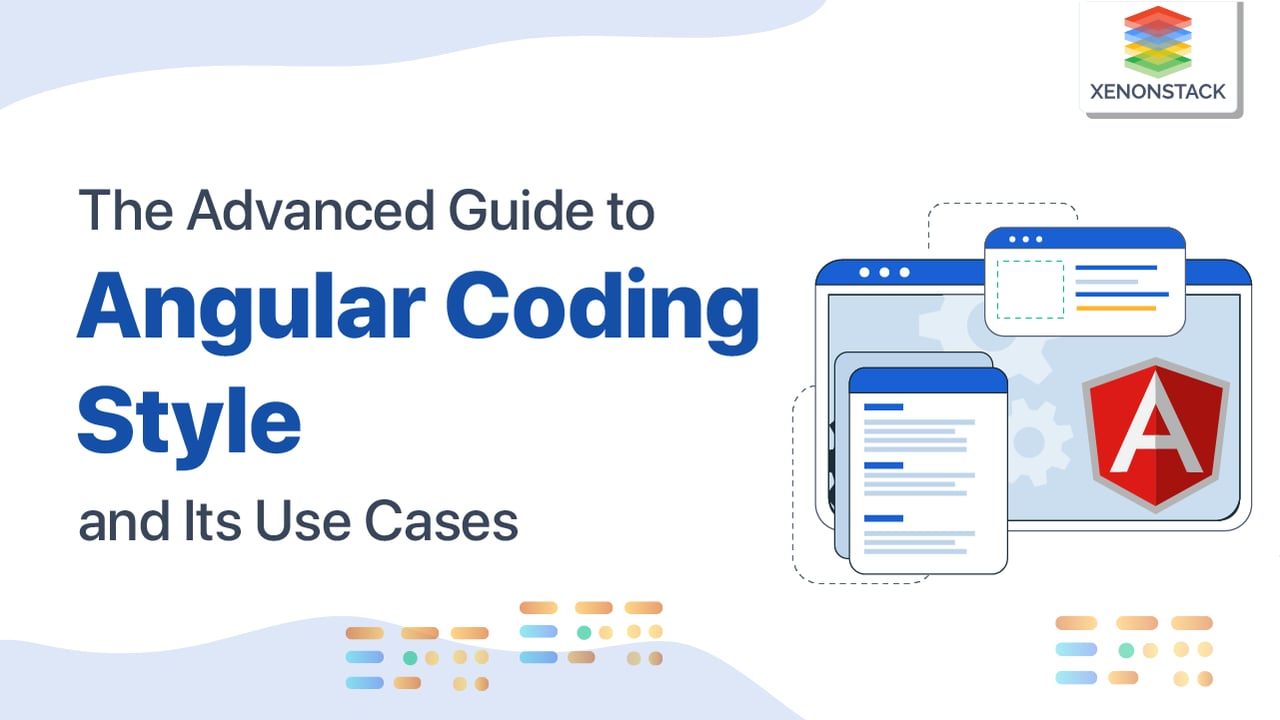
Introduction to Angular
We all know how to write code, right? The answer may be yes or no, but if you are reading this article, we are assuming that at least you know the basics of programming.
So as we all know how to do coding, why are we unable to write perfect code?
The answer is simple: we do not follow best practices, right? So what if we follow the best practices? The answer will be a great asset for our team. So here in this article, we are going to see the best practices following which we can write easily scalable and maintainable code.
What is a Style Guide?
A style guide in the context of angular is a document that contains rules about what is to be done and what is not while writing code.
A technique in which a class receives its dependencies from external sources rather than creating them. Click to explore about our, Angular Dependency Injection
Why do we use the Style Guide?
Let us consider the piece of code given below.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-parent',
templateUrl: './parent.component.html',
styleUrls: ['./parent.component.css']
})
export class ParentComponent implements OnInit {
constructor() { }
ngOnInit(): void {
}
//this function work on click
onclick(){
return "function works";
}
}
Here we have used comments to describe the function. Also, the function working is understood by the name itself. So here comes our style guide to inform us about what best practices we should follow in these cases.
For instance, for the given piece of code, we should use a function name such that it should be understood by reading what this function will do.
And for this case, there should be no use of comments.
Style Guide Methods
There are different methods in which angular style guides are used, and we will look into each method for different topics in the use case section.
What are the Use Case?
The use cases of Angular coding style are:
Do define small functions
The function should not be more than 75 lines. Because small functions are easy to understand and debug, they should also be manageable because we all know that functions come in memory when they are called somewhere.
So to utilize less memory, we make our functions, but function takes a lot of time to come to memory. Then they execute, so for small functions, the memory consumption does not matter, but they take the time to come to memory and become a curse so that we can use the concept of inline function.
Naming Rules
To write meaningful code, it is essential to write the code so that variable and function names are easily understood by other developers working on that project.
A name should be such that there is no need to write comments for a function or a class.
For giving the file name, we should use conventions for components .component, for service .service, for module .module, and if the file name is more prominent should be separated by a hyphen.
- Name for components class: If we have a class of heroes, the class name should be Hero.
Component.ts,Hero.Component.html, Hero.Component. css or scss if we are using that. And if in case we have a letter called AllHeroes, we will write with a hyphen All-Heroes.component.ts, etc. - Name for service class: If we have a hero service, the name should be HeroService.The service name should be like Hero.service.ts.
- Name for component selector: Use dash case for naming selectors. For example, if we have a hero component, we should use <app-hero selector></app-hero selector>.
- Name for module file: Name the module file with .module extensions. For example, if we have a Main module, the name should be main.module.ts.
Application structure and NgModules
Structuring files is most important for all developers, and we have some parameters that should be considered.
- Lift: Lift provides a consistent structure that scales well, is modular, and makes it easier for developers to file the code quickly.
- Locate: locate the files in a straightforward way to find them. For utility files, make a particular folder utility and file name as utility.ts
- Identity: Do name the file in such a way that what it contains should be easily understood by the one who is going to use it. Each file should have a unique name, and a component should have a similar pattern all file names should be the same, but the extensions should be different.
- Folder-By-Feature: Do create folder names for the feature areas they represent. By reading the folder name, it should be understood what will be in this particular folder.
- Lazy-Loaded-Folder: Do create a lazy-loaded folder. A lazy loading is done when we want some feature to initiate on demand rather than automatically loading at the initial state. Do put lazy loaded features in a lazy loaded folder, such as routing components, child components, and assets.
- Not to add sorting and filtering logic to pipes: Do not add sorting and filtering logic to pipes because the logic is heavy and can affect the user experience.
Component
- Component as an element: Always give the component as an element selector rather than giving it a name as an attribute or class selector. Always use <app-main></app-main> instead of adding [app-main] in the selector.
- Write templates and styles to their files: Always write them to their files because it makes our code clean, maintainable, and readable.
Don't Do This if the Html code is large.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: `<body>
<header></header>
<main></main>
<footer></footer>
</body>`,
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(){}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta HTTP-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<header></header>
<main></main>
<footer> </footer>
</body>
</html>
If your code is large, we must use an external template like the above.
- Use input and output decorator: Use input and output decorators in place of input and output of component and directives metadata property. Input and output decorators are used for sharing the data between parent and child components.
import { Component, Input, Output } from '@angular/core';
import { EventEmitter } from 'stream';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
@Input() receiveFromParent='';
@Output() sendFromChild=new EventEmitter();
constructor(){}
}
- Member Sequence: Use member sequence-first put the public properties, then private properties, and then methods—member sequence results in improved code readability.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public firstVariable?: string;
private second variable?:string;
constructor(){}
main=(value:string)=>{
this.firstVariable=value;
}
}
- Add complex logic to the service, not components: Components should be made for user interaction, and fixing user business logics complex tasks like http calls, etc., should be done by service. We make our component lightweight by making all http tasks and data-related tasks in a service.
import { Injectable } from "@angular/core";
import { HttpClient } from '@angular/common/http';
@Injectable({providedIn:'root'})
export class AppService{
constructor(private HTTP:HttpClient){
this.http.get("http://localhost:4200/users");
}
}
- Do not use event names starting from on: events are also assigned with one prefix so that they will mismatch.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta HTTP-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick= "onButtonClick()">function here made with name on button click is not a best practice</button>
<button onclick="clickOnButton()">the given function name is a best practice</button>
</body>
</html>
- Put presentational logic in component ts file, not html file: Alway put presentational logic in ts file because it will improve code testability and maintainability.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta HTTP-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick="clickHere()"></button>
<!-- the following code is not a best practice -->
</body>
</html>
Directives
- Use directives to enhance an element:
import { Directive, HostListener } from "@angular/core";@Directive({
selector:['toColor']
})
export class ColorDirectives{
@HostListener('mouseover') on mouse enter(){
//do some task
}
}
Services
Provide a service: Do provide a service with @injector decorator and define an object {providedIn:’for-root'}.
import { Injectable } from "@angular/core";
import { HttpClient } from '@angular/common/http';
@Injectable({providedIn:'root'})
export class AppService{
constructor(private HTTP:HttpClient){}
}
Talk to a server threw a service
Always use the service for calling HTTP requests. it makes the task easy for components to do the main work. As the above example indicates, we have used the HttpClient module to communicate between the client and server computer.
Conclusion
Through this blog, we have come to know the following points.
1. Style guide tells us how to write clean and maintainable code in angular.
2. Naming conventions should be followed while writing file and folder name, service name , module names, pipe names, etc.
3. Use of directives should be done to enhance the element.
4. Use of service should be done to perform complex tasks, and components should deal with doing business logic.
5 . Service should be used to call HTTP requests to the server computer.