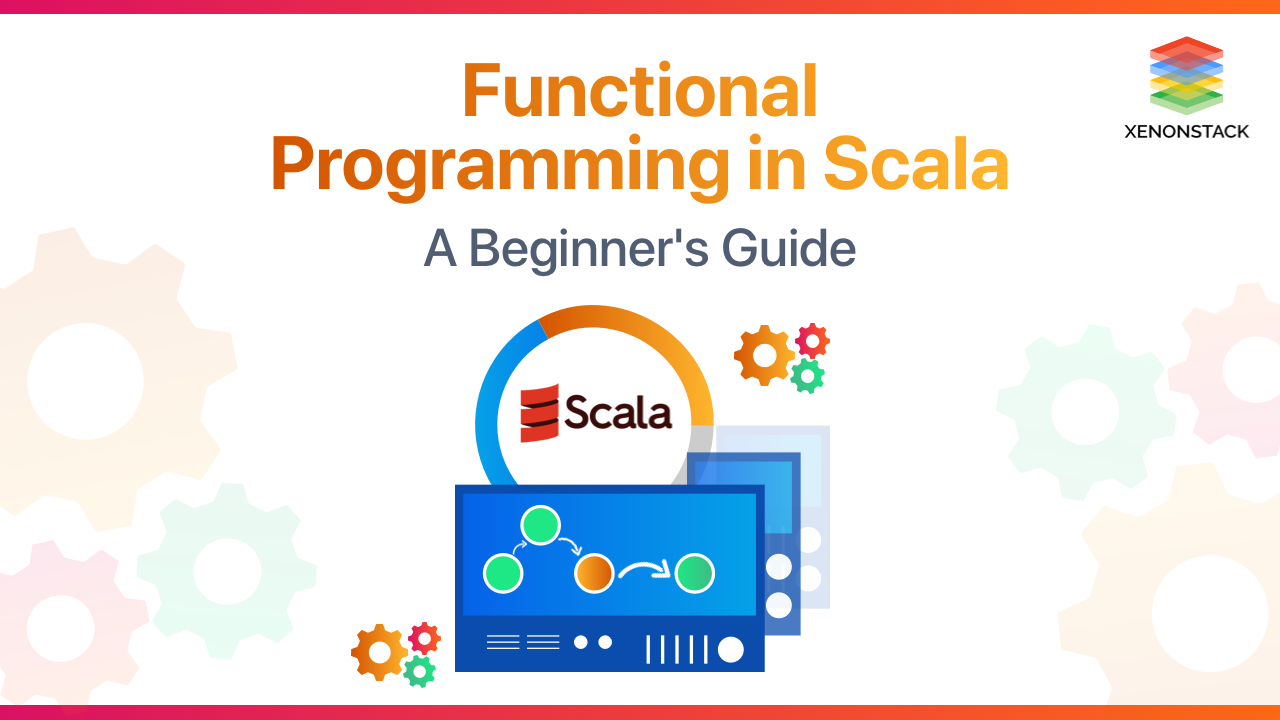
Overview of Functional Programming in Scala
Scala is a modern, statically typed, general-purpose, high-level programming language supporting object-oriented and functional programming. It was created and developed by Martin Odersky and started in 2001. The name scala comes from the word Scalable, and it is used by most of the famous engaged sites like Netflix, Twitter, Linkedin, and many more.
The Key Features of Scala are :
- It is Object-Oriented
- It is Functional
- It is statically typed
- It is a High-level programming language
- It lets you write concise and readable code
- It runs on Java Virtual Machines
- It works well with most of the Java libraries
Functional Programming is a way of writing applications using only pure functions and immutable values.Taken From Article, Functional Programming: Why is it Liked so much by the Developers?
What is Functional Programming?
Functional programming is the paradigm of developing software with the use of pure functions and immutable values. It is Declarative in style, avoiding a shared state. Declarative programming is about writing code in a way that describes what you want to do by using a set of declarative statements, each of which has a meaning and can be understood independently. In Functional Programming, functions are treated as First-class citizens where they can be assigned names, passed as arguments, and returned from other functions as data types.
Pure Function
A pure function is a function
- Whose output depends only on the input values and it’s implementation.
- On passing the same input files, it will always return the same values
- It does not read data from the outside world
- It does not mutate the hidden state.
Immutability
Immutability is unable to change value. It is essential as if you want to benefit from your multicore CPU and run your code parallelly, immutable fields ensure that no thread accidentally changes the value of the field. Immutable objects are safer while running multi-threads.
Scala is essentially the C++ of high level (ergo, memory managed) languages on the JVM. Taken From Article, Scala Programming Language
How is Scala Functional?
Scala is functional in the sense that each function is a value in scala. In Scala, functions are first-class citizens who can be assigned names. It also supports currying and anonymous functions. Hence allowing programmers flexibility to write clean, concise, and elegant code.
What are the various types of Functions?
The various types of functions are listed below:
Normal Function
Writing pure functions in scala is easy, normal method syntax can be used to write a normal pure function. Check out the following example that adds 2 to the number passed-in function.
def double(i: Int): Int = i + 2
Functions that accept Functions
Scala provides the capability to pass functions as arguments in other Function. For example, in the following example, multiply function is taking a function as an argument where triple function has been passed to multiply the digit with 3
object Multiply extends App {
def double(i: Int): Int = i * 2
def triple(i: Int):Int = i * 3
def multiply(value:Int, mulFunc : Int => Int) = mulFunc(value)
println(multiply(3,triple))
}
Setting up Continuous Delivery Pipeline for Continuous Integration and deployment of Scala Microservices application with Jenkins, Docker and Kubernetes.Taken From Article, Continuous Delivery Pipeline for Scala Application on Kubernetes
Functions that return Functions
Scala provides the capability to return functions from functions. For example, the below function Greetings function returns a function to return Hello based on language passed.object Hello extends App {
//Greetings function as a main wrapper to select language and greet
def Greetings(lang: String): String = {
lang match {
case "spanish"=> GreetingsSpanish()
case _ => GreetingsEnglish()
}
}
//Greet in Spanish
def GreetingsSpanish():String = "Hola"
//Greet in English
def GreetingsEnglish():String = "Hello"
//Call greetings function
println(Greetings("spanish"))
}
Developing Android applications is a great option to drive success to your business but, picking up the best programming language is the real dilemma.Taken From Article, Kotlin vs Java: Which is Better for Android App Development?
Currying Functions
Currying Functions are functions that take multiple arguments to execute statements.
object Hello extends App {
def Add(i:Int)(j:Int):Int =i+j
println(Add(2)(3))
}
Anonymous Functions
A function which does not have a name is called an Anonymous Function. It can also be called a Function Literal. It can be used where inline functions are required. object Greetings extends App {
val numbers = List(1,2,3)
val modifiedNumbers = numbers.map((i:Int)=>i*2)
println(modifiedNumbers)
}
Closures
Closures are functions that use one or more free variables, and the output of these functions is dependent on these variables. The free variables are declared outside the Closure. Closures can be pure if free variables being used are immutable, neither function’s code nor other code can change its value.object Hello extends App {
val p = 10
def double(): Int = p * 2
println(double)
}
Recursive Functions
Recursive functions are the functions that keep calling themselves until a break condition is reached. object Greetings extends App {
def fact(num:Int): Int={
if(num == 1) 1
else num * fact(num - 1)
}
println(fact(3))
}
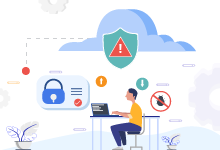
Conclusion
Programming in a Functional way helps to ensure brevity in the code. It also allows one to implement concurrency safely and take advantage of your multi-core CPU. Scala being a Multi-Paradigm Language, will enable one to code with both Object-Oriented and Functional programming approaches at the same time in an elegant way. All these powerful features make Scala a suitable language to opt for Functional Programming.
- Explore the comparison between Golang vs Rust
- Discover more about Functional Testing and Its Types