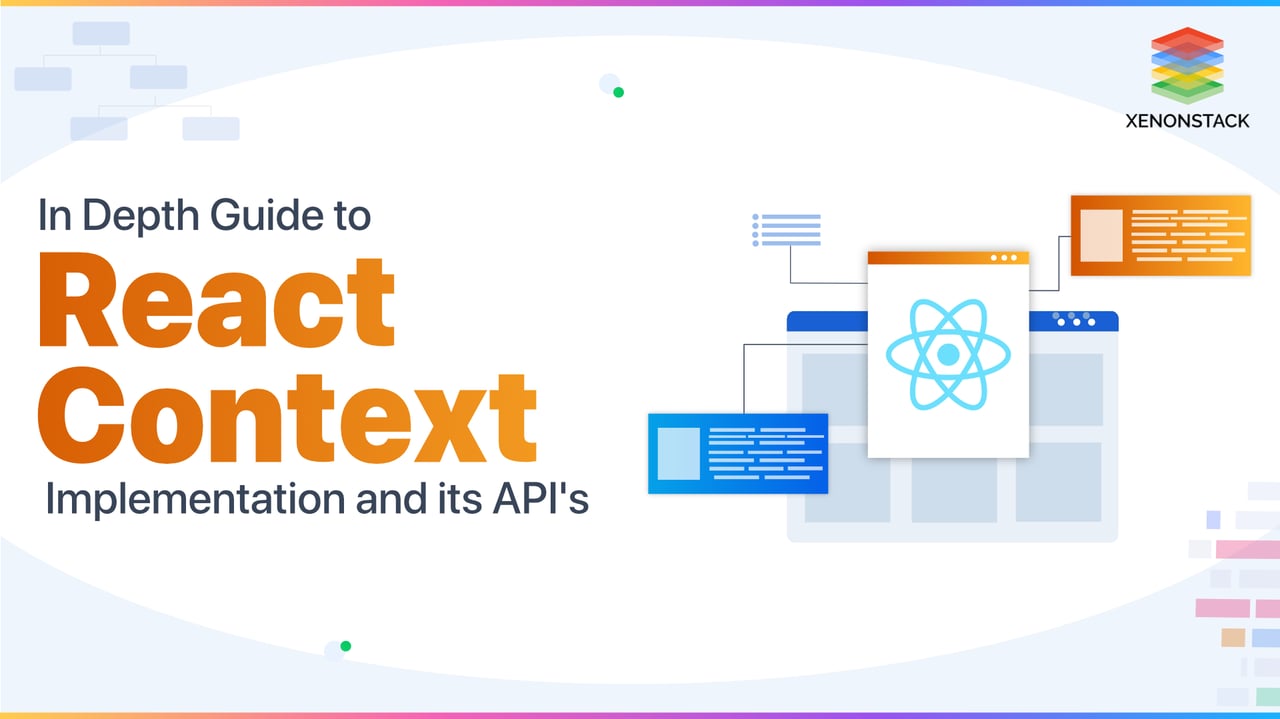
Introduction to React Context
Context allows you to transfer data down the component tree without explicitly passing props at each level.
Props are used to send data top-down (parent to child) in a typical React application, but this might be inconvenient for certain props (e.g. locale preference, UI theme) required by multiple components within an application. Context allows components to share values like these without passing a prop through each tree level directly.
ReactJs Project Structure with best boilerplate structures that most of the react community prefers. Click to explore about, ReactJs Project Structure and Folder Setups
What is React Context?
When sending data that any component in your application may utilize, React context is ideal.
The following are examples of data types:
- Theme information (like dark or light mode)
- Information about the user (the currently authenticated user)
- Data specific to a particular location (like user language or locale)
- Data should be stored in a React context that does not require frequent updates.
Why? Because the context was not created as a full-fledged state management system. It was created to make data consumption easier.
React context can be thought of as the equivalent of global variables for our React components.
What is the use of context Hook?
The render props pattern for consuming context may appear foreign to you. With React hooks in React 16.8, a new technique of consuming context became accessible. With the use of the context hook, we can now consume context. We can give the complete context object to React instead of utilizing render props. At the beginning of our component, we use context() to consume context. The use context hook is used in the following example:
A process of analyzing the performance of web applications with all their components. Click to explore about, How to Profile a React Application?
Why React Context is important?
We can avoid props digging by using React context. Props drilling refers to passing props down multiple levels to a nested component via components that don't require it. This is an illustration of prop drilling. We have access to theme data in this app, which we want to send as a prop to all of our app's components. We're forcing the theme prop into several components that don't require it right away.
The Header component only needs the theme to send it down to its child component. In other words, it would be preferable if the User, Login, and Menu could directly consume theme data. This is one of the advantages of React context: we can completely avoid utilizing props and avoid the problem of props drilling.
When to use React Context?
When numerous components at various nesting levels need access to the same data, context is employed. It should be used with caution because it makes component reuse more difficult. Component composition is typically simpler than context if you merely want to avoid providing some props over several layers.
A process which is used for increasing the quality of a software or a product and for improving it by identifying defects, problems, and errors. Click to explore about, Javascript and ReactJS Unit Testing, TDD and BDD
How to implement React Context?
With React version 16, Context is an API incorporated into the framework. By importing React into any React app, we may construct and use context immediately.
React context is implemented in four steps:
- Using the createContext method, you may make a context.
- Wrap the context provider around your component tree using the context you just built.
- You can assign any value to your context provider using the value prop.
- Using the context consumer, you may find that value in any component.
Let's look at a simple illustration. Let's use Context to pass down 'Hello', which we'll read in a nested component called User in our app.
What is the Context API in React?
The React Context API are listed below:
React.createContext
This method creates a Context object. React will read the current context value from the closest matching Provider above it in the tree when rendering a component that subscribes to this Context object.
When a component doesn't have a matching Provider above it in the tree, the defaultValue parameter is applied. This default value can be useful for testing components without enclosing them in a container. Note that supplying undefined as a Provider value does not force defaultValue to be used by consuming components.
Context.Provider
A Provider React component is included with every Context object, allowing consuming components to subscribe to context changes. A value prop is supplied to consuming components that are descendants of this Provider by the Provider component. Many consumers might be connected to a single Provider. To override values farther down the tree, providers can be nested.
When a Provider's value prop changes, all consumers who are descendants of that Provider will re-render. The shouldComponentUpdate method does not apply to propagation from Provider to descendant consumers (including.contextType and useContext). Therefore, the consumer is updated even if an ancestor component skips an update.
An asynchronous programming model where the developer process the stream of coming data to propagate the changes in code. Click to explore about, Reactive Programming Solutions for Monitoring Platform
Context.Consumer
A React component that listens for changes in the context. You can subscribe to a context within a function component using this component. It is necessary to perform the function of a child. The current context value is passed to the function, which returns a React node. The value prop of the closest Provider for this context above in the tree will be provided as the value argument to the function. The value argument is equivalent to the defaultValue supplied to createContext if there is no Provider for this context above ().
Context.displayName
A display name string property is available on the context object. React DevTools use this string to identify what should be displayed in the context. The following component, for example, will appear in the DevTools as MyDisplayName:
What is Context in React with example?
The React Context is explained below with examples:
Consuming Multiple Contexts
We may wish to include several contexts in our programmes, such as using a single theme for the entire app, altering the language based on the user's location, A/B testing, and using global settings for login or user profiles. As in the code below, we created two contexts ThemeContext. We passed a string for the current context, 'light', which means the current theme for a component is Light, and another context if UserContext, which contains property as name with value 'Guest'.
Serving 'n' number of contexts in the "m' component and keeping the changed value in each rendered component is doable in React, although this significantly slows down rendering. To avoid this and make re-rendering faster, each context consumer in the tree should be treated as a separate node or divided into different contexts. So we have our two Context objects. Now we will make both contexts available to all our components. We will use Provider as used in the below code:
At last, we will store the value/information by using Consumer. Here if the parent component 'ThemeContext' context value changes, the child components' UserContext' or components' ProfilePage' that hold that value should be rerendered or modified. As a result, anytime the value of a provider changes, it will trigger its consumers to re-render.
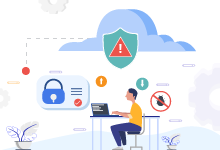
Conclusion
The following are the primary takeaways from this topic :
- The React Context API was created with prop digging in mind.
- If you're going to use Context for global state management, be careful.
- Try Redux if you can't be careful with Context.
- Redux is not the only state management technology available; it may be used without React.
We went over the React Context API, when we should use it to avoid prop drilling, and how to use it most efficiently in this topic. We also dispelled some common misunderstandings about the React Context API.
- Click here to learn React | Advanced Guide for Angular Developers
- Discover more about Optimizing React Application Performance