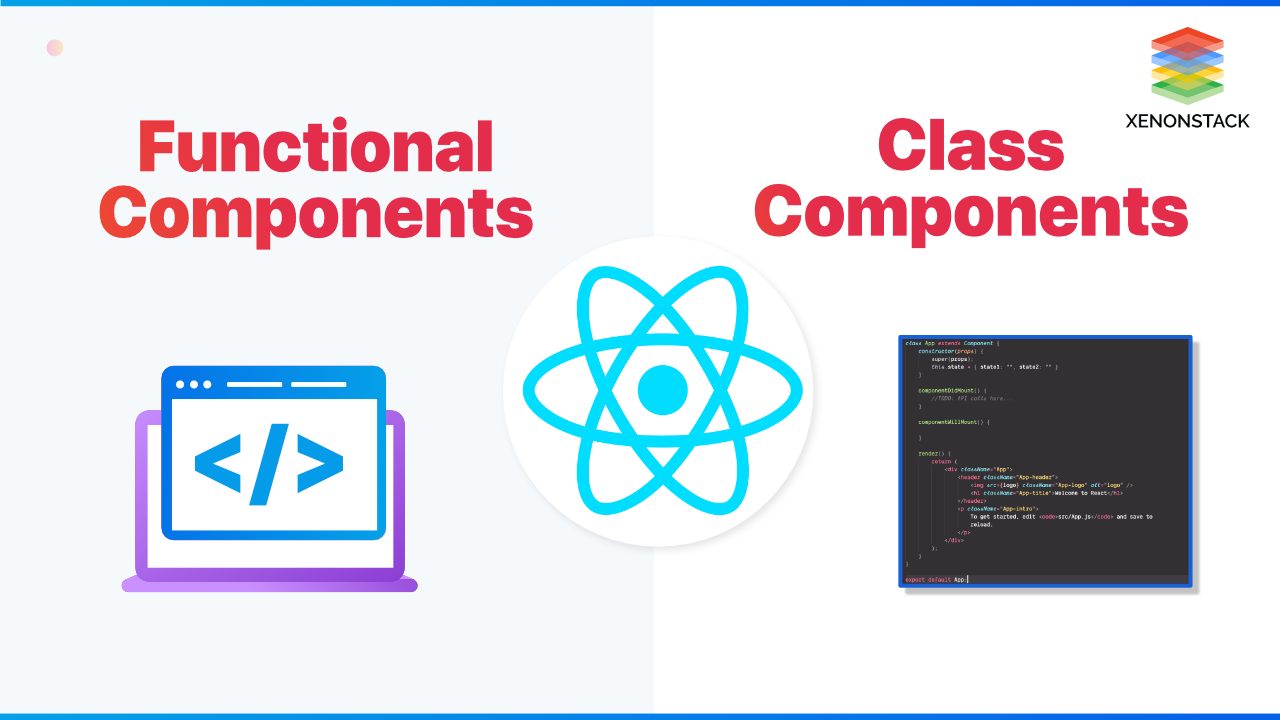
Introduction to Functional and Class-Components
Developing a single page application, developers have to write more than thousands of lines of code that follow the traditional DOM structure. If any changes are needed that make it challenging to updation, a component-based approach is introduced to overcome this issue. In this procedure whole application is divided into a logical group of code, which came to know as Component.
ReactComponent is considered as a brick of a wall. It makes the building UI lot easier. Final UI depends on which components are in use, and they all merge into a parent component.
It provide its primary function through Components that are re-usable and independent bits of code. Components are similarJavascript functions but work as isolated and return HTML.
A process of analyzing the performance of web applications with all their components. Click to explore about, How to Profile a React Application?
What is React Component?
They have their structure, whereas its developer team added more features like libraries or tools for coding. It offere two types of components for creating UI components. Both components provide similar functionalities, and the choice only depends on the developer’s preference. Here we can slightly differ the previous line by describing a difference; the actual situation was that only Class components were the only viable option to develop a complex app with it.
The reason behind this is while using class components. For example, we have many usability features, while it do not provide such an option. But after React v.16.8 was released, it contained an update that was meant to take further development levels, Here it offered Hooks for it. Using hooks, it made possible to write complex applications using only functions as its components.
Let’s find out the features in the present time.
React server Component works to re-write its components that are rendered on the server-side to enhance application performance. Click to explore about, Server Components Working
What is Functional Components?
In reacting, they are just functions of Javascript functions like this:
function Sample(props) {
return hello world! Reading this blog? - {props.name};
}
const ele = <Sample name="Me!" />;
ReactDOM.render(ele, document.getElementById('home'));
In the above case, we render a user-defined component Sample. The element as ele passes JSX attribute name= ”ME” as a prop to the function Sample, which returns an < h1 > element as a result. For both types of components, props are inputs.
After importing it, we call this component like this :
import Sample from './sample';function App() {
return (
<div className="App">
<Sample />
</div>
);
}
So it must return JSX, Starts with Capital letter & follows pascal naming convention, takes props as a parameter if needed.
React 16 presented a new concept of an “error boundary” to solve a problem when a part in the UI contains a JavaScript error. Click to explore about, Error Handling in React 16
What is Class Components?
There are additional features of these components, like maintaining logic and state. That's why it is called stateful or intelligent components.
- You can use its lifecycle methods throughout the component like componentWillMount etc.
- In components, we can access props pass down like this. props.
- Class components make use of ECMAscript or ES6 class, then extend the component class.
class Checkyourscore extends React.Component {
render() {
return Hello! I'll calculate your marks!😎 {this.props.name};
}
}
It is an ES6 class that extends class components from its library. Here we used render() to return HTML.
Class components work fine with props. You can use a syntax similar to an HTML attribute using props to bypass a component. In the above example, we need to replace props.name with this.props.name in the render() body to use props. The state of a component has to initiate this.state that can be assigned with a class constructor.
Using React in application brings better performance and minimizes the number of DOM operations used to build faster user interfaces. Click to explore about, Optimizing React Application Performance
Difference between Functional and Class components?
The complete comparison is described below:
State and lifecycle
In this section, we will differentiate based on features as that class provide devs with such functionality as lifecycle and setState(). Methods like componentWillMount, componentDidUpdate, etc., while functional component doesn’t because these are simple JS functions that accept props and return its elements. In contrast, the class component has a render method that extends its Component. However, it had changed when Hooks came to the introduction. Component lifecycle classes have methods like componentDidUpdate. The component will unmount, shouldComponentUpdate. They have a tool that works the same as mentioned methods using only one Hook useEffect. So Hooks are like an addition for it, rather than a replacement of class components.
Syntax
There are different types of the syntax used in both components. Let’s look into some examples.
Declaring Components
- Functional components are JS functions:
function FunctionalComp() {
return <h1>Hello, sup world</h1>;
}
- Class components are classes that extends React.Component
class ClassComp extends React.Component {
render() {
return <h1>Hello, sup world</h1>;
}
}
Fot return <h1> tag we use render() method inside class component.
Passing Props
For example, there is a prop named: “Pickone”.
<component name = ‘First’ />
While using the functional component, we pass props as an argument of our function. “props.name” construction.
function FunctionalComp(props) {
return <h1>Hii, {props.name}</h1>;
}
While in-class class component, we add this. to refer props.
class ClassComp extends React.Component {
render() {
return <h1>Hii, {this.props.name}</h1>;
}
Handling State
For handling state in functional component React offer useState() Hook . let’s describe it using an example there is functionality added whenever we click it increases the count for this initial state of count set to 0 using method setCount() that increase the count every time click on the button .
const FunctionalCount = () => {
const [count, setCount] = React.useState(0);
return (
<div>
<p>count: {count}</p>
<button onClick={() => setCount(count + 1)}>Click this button</button>
</div>
);
};
We use the setState() function in the Class component, which requires a constructor and this keyword.
class ClassCount extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
render() {
return (
<div>
<p>count: {this.state.count} per times clicked</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Click this button
</button>
</div>
);
}
}
Here, both components have similar logic. State object, state key ‘count’ and initial value declared under constructor(). In render(), we use the function to update count value using this.state.count, and it renders the count of the button clicked also displays the button. Results are the same, but all the difference is that lines of code require more in-class components.
Hoisting only for functional components
According to the Hoisting concept, JS moves function and variable declaration to the top to access a variable or function, which allows access first, then declares it. While during the compile phase, it puts a declaration in memory that allows the calling function before declaring it. To get access to a class before the declaration throws a ReferenceError exception in classes.
Let’s look into an example where we call the function before its declaration:
petName(‘Ruby’);
function petName(name) {
console.log(‘My pet’s name is ’ + name);
}
// The result is: ‘My pet’s name is Ruby’
The above code will work fine, but it will get a reference error while declaring a class.
Like
const p = new MyName(); // ReferenceError
class MyName {}
Or we declare a variable and call it before the initialization returns undefined >
console.log(myName); // Returns undefined, as only declaration was hoisted
var myName; // Declaration
myName = “John”; // Initialization
Here, initialization with const and let are hoisted but not initialized means our app is aware of the existence of variables.
If we use a simple component that only returns a statement, there is no need to separate it and merge it into the root index.js file.
Performance difference
In this section, each developer might not consider this because it all depends on the usability of the developer. It shows a greater performance than class components. The point used to measure this is made of a simple object with the type(string) and props(object) 2 properties. Rendering such a component needs to call the function and passing props.
Class components are more complex because they are the instances of it. Component with constructor and complicating system of methods of using and manipulating state and lifecycle. In brief, theoretically, we can say calling a function takes less time compared to creating an instance of a class.
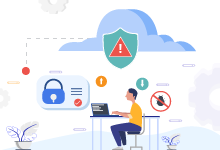
Conclusion
It all depends on the application, whether it is complex or not. The developer decides according to the requirement if it is less complex, then moves forward to the functional component, but when hooks become known, it erases approach all differences. Also, it depends on code readability, where functions seem very friendly to use.
- Discover more about React.JS Application Development Services
- Click to explore Reactive Programming Solutions for Monitoring Platform