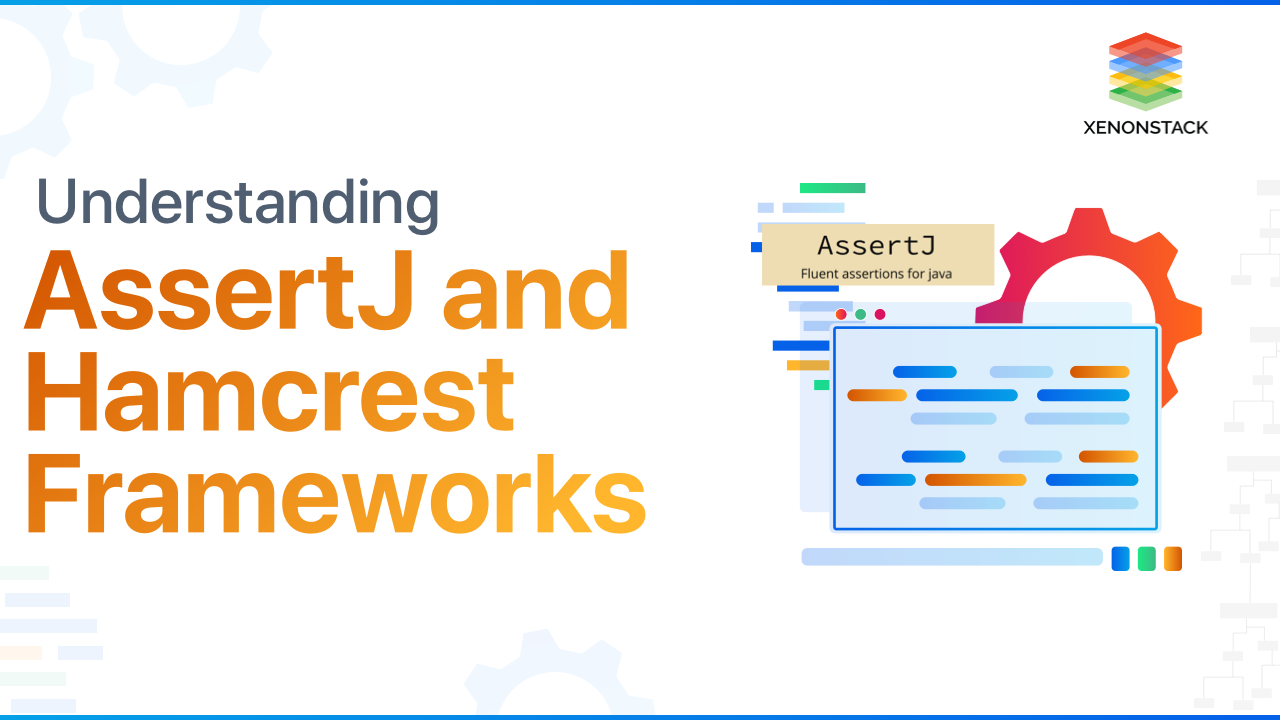
What is AssertJ and HamCrest?
With assertions, validate if something is working correctly or not, one can validate the response to check the functionality of the component. There are two of the most popular Java assertion frameworks which are available in the market and also these are open source. AssertJ is Java library that provides an interface for writing assertions. Test code readability and make maintenance of tests easier are the main goals of it.
Capsule Network is just a different way to implement neural network. Click to explore about, Capsule Networks Best Practices
It is not as much popular as Hamcrest, but its popularity has been growing very fast in the last few years. It is one of the well known Java frameworks used for writing tests used for writing matcher objects, which are logically equal to "assertions." Due to its high popularity, later ported to other programming languages like C++, C#, Python, ActionScript 3, Objective C, PHP, JavaScript, R, and Erlang.
How does it Work?
The working process is described below:
Add below dependency in your maven project in a pom.xml file.
org.hamcrest
java-hamcrest
{version}
test
Basic example -
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
import junit.framework.TestCase;
public class TeaTest extends TestCase {
public void testEquals() {
Tea yoursTea = new Tea("Ginger");
Tea myTea = new Tea("Ginger");
assertThat(yoursTea, equalTo(myTea));
}
}
Syntax -
// Simple Assertions
assertThat(a, equalTo(b));
// Dates Assertions
assertThat(tomorrow, isAfter(today));
// List Assertions
assertThat(list, Matchers.<collection> allOf(CoreMatchers.hasItem("a"),
CoreMatchers.not(CoreMatchers.hasItem("b"))
));
</collection
// Null Assertions
assertThat(a, nullValue());
// Assertions With a Custom Message
assertThat("Error", a, equalTo(b));
Getting Started with AssertJ and HamCrest
Add below dependency in your maven project in a pom.xml file.
org.assertj
assertj-core
{version}
test
Syntax -
// Simple Assertions
assertThat(a).isEqualTo(b);
// Dates Assertions
assertThat(tomorrow).isAfter(today);
// List Assertions
assertThat(list).
contains("a").
doesNotContain("b");
// Null Assertions
assertThat(actual).isNull();
// Assertions With a Custom Message
assertThat(a).isEqualTo(b).overridingErrorMessage("Error");
AssertJ and Hamcrest Examples
Below are some examples which show the similarities and differences of the common assertions between both -
// Equality
assertThat(actual, equalTo(expected)); // Hamcrest
assertThat(actual).isEqualTo(expected); // AssertJ
// Null checking
assertThat(actual, nullValue()); // Hamcrest
assertThat(actual).isNull(); // AssertJ
// Boolean checks
assertThat(actual, is(true)); // Hamcrest
assertThat(actual).isTrue(); // AssertJ
// Double comparisons
assertThat(actualDouble, closeTo(expectedDouble, error)); // Hamcrest
assertThat(actualDouble).isCloseTo(expectedDouble, Offset.offset(offset)); // AssertJ
// Assume/Assumption (JUnit’s skipping behavior)
assumeThat(actual, equalTo(expected)); // Hamcrest
// n/a AssertJ
// Expect (fail-at-end)
// n/a Hamcrest
softly.assertThat(actual).isEqualTo(expected); //AssertJ
What are the benefits?
There are some benefits of these Java assertion frameworks which are defined below -- Quality of code
- Easy to find bugs
- Facilitates changes
- Simplifies Integration
- Debugging
Explore the process of how you can Develop and Deploy Microservices based Java Application on the Container Environment – Docker and Kubernetes and adopt DevOps in existing Java Application. -From the Article,Java Microservices Application Deployment on Kubernetes
Why it is imporatant?
There are many key points which explained that why these frameworks matters.
Readability
Both frameworks feel more natural and are easily readable and understandable as compared to others. Let's take an example of assertion.
assertEquals(expected, actual);
The above is a common way, the expected value is passed as the first argument and the actual value is passed as the second argument. But this method is very confusing until one can get used to it. But in the case of these two frameworks, the actual value is passed the as the first argument and the expected value is passed as the second argument, which is the biggest hurdle to get over.
assertThat(actual, equalTo(expected)); // Hamcrest
assertThat(actual).isEqualTo(expected); // AssertJ
Better Failure Messages
Much better error messages. In most of the other frameworks, the problem is that the assertion error does not report the expected values and actual values. Let's see an example -
assertTrue(expected.contains(actual));
java.lang.AssertionError
It doesn't provide the clearance of the error message but below code snippet provides a better explanation of an error which saves the time and hassle of a developer.
assertThat(actual, containsString(expected));
java.lang.AssertionError
Expected: a string containing abc
got: "def"
Custom Messages
Can also use assertions with custom messages in case of them. Can also pass any user-defined message. Let's take an example -
assertThat("Error", a, equalTo(b)); // Hamcrest
assertThat(a).isEqualTo(b).overridingErrorMessage("Error"); // AssertJ
Portability
In case of it, portability is also the advantage of its library. It can be used with both JUnit and Testing. Type Safety - Another advantage of these assertThat is that it is type-safe. It does not allow comparing of different types. Let's take an example -
assertEquals("abc", 123); //compiles, but fails
assertThat(123, is("abc")); //does not compile
How to adopt it?
Add the dependency according to the particular framework in pom.xml file.// Hamcrest org.hamcrest java-hamcrest {version} test
// AssertJ org.assertj assertj-core {version} test
Import dependencies
Below are the basic examples -
// Hamcrest
@Test
public void hamcrestTestExample() throws Exception {
// Given
Integer number = 5;
// Then
assertThat(number, greaterThan(3));
}
// AssertJ
@Test
public void assertJTestExample() throws Exception {
// Given
Integer number = 5;
// Then
assertThat(number).isGreaterThan(3);
}
Concluding Assertion Frameworks
Hamcrest is much more popular as compared to AssertJ. But when we compare by commits, it has a more stable development pattern in the last years. On comparing the both Google Trends found that it is much more popular. The primary purpose is to tell about the most popular Java assertion frameworks available in the market. Can use any one of these two frameworks, to extend the capabilities of the standard assertions use any of the frameworks between these two. Each programming language has its libraries for assertions which help users to implement different kinds of assertions easily.
- Discover more about Open Source Big Data Tools and Frameworks
- Explore more about Integration of dot js Frameworks
- Read here about Software as a Service Testing Tools