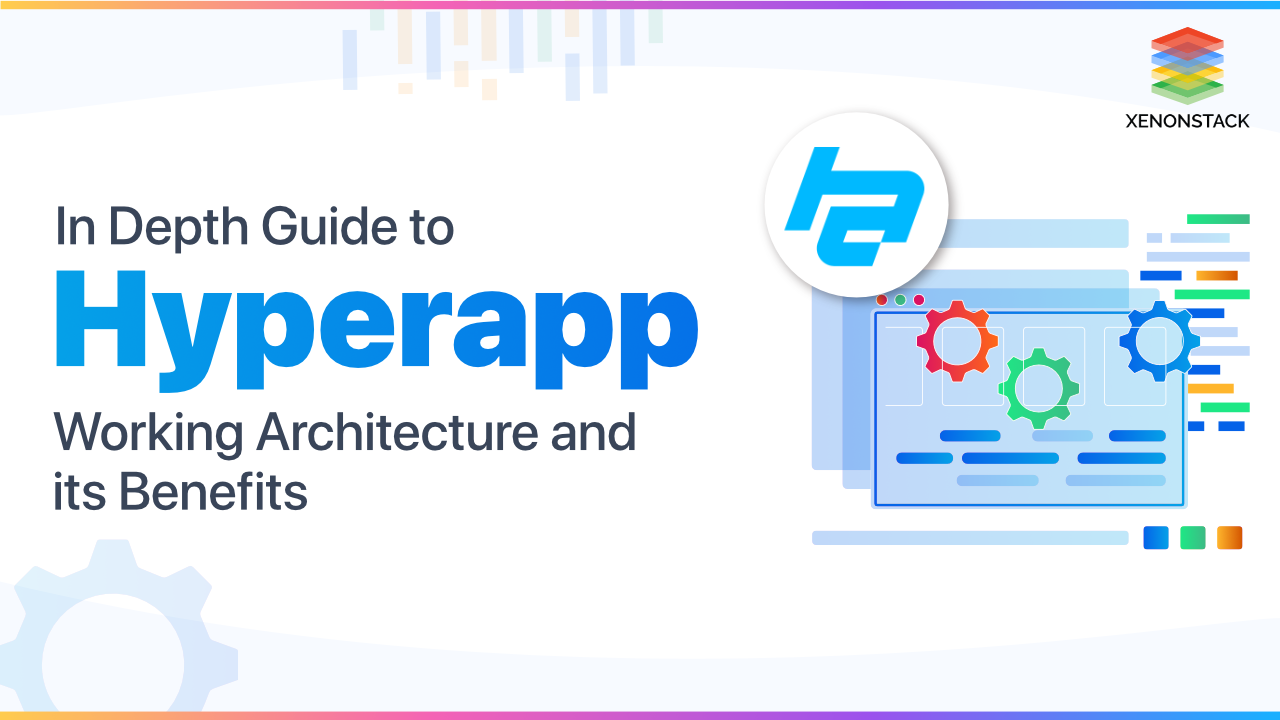
What is Hyperapp?
Hyperapp is a lightweight Javascript framework for developing feature-rich web applications. It is written in ES5, i.e., ECMAScript 5. Hyperapp requires Node.js, NPM, JSX, and Webpack modules to be installed on the system before jumping on building a web application. The idea behind working on the Hyper App framework is to get more from less, i.e., minimizing the dependencies and usage of simpler software code.
Compared to other frameworks like React, Preact, Vue, Ember, Mithril, etc., the HyperApp framework is a very compact API with minimal bundle size and built-in state management. It has a strong foundation and significant community support. One can participate in their online community support for developing Hyperapp, a robust frontend Javascript framework.
In JavaScript, various libraries are open source and commercial both, with which data visualization tasks can be performed easily. Click to explore about, Data Visualization JavaScript Libraries
How Hyperapp Works?
Hyperapp framework wraps up the three interconnected approaches i.e., state, actions, and view. The state updates the changes every time the user acts on the interface(view). The view is responsible for rendering the changes over the user interface, which the user can see.
An action is a unary function with a single argument expecting a payload. The payload could be anything the user wants to pass into the action. On each action call, the state changes, and then the state redraws the changes onto the view. Here is a simple "Hello World" example -
import { h, app } from "hyperapp"
const state = { title: "Hi." }
const actions = {}
const view = state => {state.title}
app(state, actions, view, document.body)
Code bounding simple HTML input -
import { h, app } from "hyperapp"
const state = {
text: "Hello!"
}
const actions = {
setText: text => ({ text })
}
const view = (state, { setText }) => (
<main>
{state.text.trim() === : state.text}
<input
autofocus
value={state.text}
oninput={e => setText(e.target.value)}
/>
</main>
)
app(state, actions, view, document.body)
The user triggers action on the web application. After the action is initiated, the action function is called and takes responsibility to replace the previous state with the new state and update the state. The state is updated asynchronously when the action is triggered, changes to the user interface (view), and the whole process follows. HyperApp includes stateless components, which are pure javascript functions that render themselves and allow actions to trigger. These functions are framework-agnostic and easy to test and debug.
What are the benefits of Hyperapp?
The benefits of Hyperapp are listed below:
- Lightweight Javascript library - HyperApp framework has a build size of 1KB (1.3 KB) delivering the purpose of building web applications with simple and easy.
- State Management— The HyperApp framework is based on Elm architecture, just like React and Redux. However, unlike React, which depends on Redux for state management, it has built-in state management. Therefore, it delivers functional web applications efficiently with fewer lines of code.
- Minimal (Simple to understand)—The HyperApp team aggressively minimizes the concepts users need to follow to be productive. Therefore, with fewer dependencies and just a hundred lines of code, it becomes easier for newcomers to adopt such an approach.
- Standalone— The HyperApp approach is to do more with less. HyperApp supports inbuilt state management with virtual DOM, which supports key updates and lifecycle events—all with no dependencies.
- Pragmatic— When managing the state, HyperApp is firm on functional programming but follows a practical approach to allow for side effects, DOM manipulations, and asynchronous actions.
- Not a one-man-effort - HyperApp has a broad foundation and supports the community which helps the framework to get better and more flexible every time.
Implementing S.O.L.I.D principles in code will help in the Code reusability, Adapt to new code or easy code changes in future, Easy to read, Easy to maintain, and Easy to test. Click to explore about, SOLID Principles in JavaScript and TypeScript
Why Hyperapp Matters?
HyperApp is lightweight, easy to use, less number of lines of code, if anyone doesn't want to write the code or wants to create the app quickly, use HyperApp. It can be a better option for smaller applications; one can not recommend it for complex applications.
Event handling and changing components' states are easier in Hyperapp than in Redux. HyperApp is easy to learn if the user is familiar with the Redux framework. Its lifecycle is similar to React's: create, update, move, destroy. API calls are easy to handle in Hyperapp.
How to adopt Hyperapp?
Here is a simple counter application to make the user familiar with working of the HyperApp framework - Make a dir named "hyperapp" on the local system and make the dir working -
mkdir hyperapp
cd hyperapp
Running any javascript application requires the package.json file.For the yarn package manager
yarn init
For the npm package manager
npm init
Once the package manager is initialized, then the package.json file will be made in the directory. Install dependency parcel-bundler in application -
yarn add --dev parcel-bundler babel-preset-env babel-preset-react
Parcel-bundler is a web application bundler that keeps all our application files - JS, CSS, and HTML in one place and makes a proper bundle. We can also use webpack instead, but this approach is most comfortable to set up. Install the HyperApp dependency in the application.
yarn add hyperapp
Or
npm install hyperapp
Make a file in the project with extension ".babelrc" and keep it in that project root path.
{
"presets": ["env", "react"],
"plugins": [["transform-react-jsx", { "pragma": "h" }]]
}
Please note, no React used here, just including "React " as an environment for "presets" and utilizing the same settings for Babel. Make two files named - >index.html
<!DOCTYPE html>
<html>
<head>
<title>Hyperparcel Starter</title>
</head>
<body>
<div id="app"></div>
<script src="index.js"></script>
</body>
</html>
This file loaded and initialized the very first time the application runs and makes the entry point in the application which is "index.js" file. > index.js
import { h, app } from "hyperapp"
const state = {
count: 0
}
const actions = {
down: () => state => ({ count: state.count - 1 }),
up: () => state => ({ count: state.count + 1 })
}
const view = (state, actions) => (
<main>
<h1>{state.count}</h1>
<button onclick={actions.down}>-</button>
<button onclick={actions.up}>+</button>
</main>
)
const main = app(state, actions, view, document.body)
Make sure to keep both the index.html and index.js files in the root path of the project. Launch the application and run the development server. Run the Parcel command, but the Parcel not included in the bin path, include start and build scripts under the scripts section in the package.json file.
{
"name": "hyperapp",
"version": "1.0.0",
"description": "A starter for building apps with Hyperapp and Parcel.",
"main": "index.js",
"scripts": {
"start": "parcel index.html",
"build": "parcel build index.html --public-url ./"
},
"repository": "https://github.com/cutemachine/hyperparcel.git",
"author": "jo@cutemachine.com",
"license": "MIT",
"devDependencies": {
"babel-preset-env": "^1.6.1",
"babel-preset-react": "^6.24.1",
"parcel-bundler": "^1.5.1"
},
"dependencies": {
"hyperapp": "^1.0.2"
}
}
Now the package.json file will look similar to this - This file includes -
-
The name consists of the name of the project, "hyper app"
-
version includes the version of the project.
-
A description of the project (not compulsory) is included.
-
Central includes the main entry of the project, which is the "index.js" file.
-
Scripts include the start, build and other scripts required when building and starting the application.
-
The repository consists of the git repository where our project is present, and anyone can clone this repo in a local system when running this project.
-
The author is the name of the developer who has to build the project.
-
The license includes the standard "MIT" license provided.
-
DevDependencies includes all the development based dependencies which are required at the time of development.
-
Dependencies include all the dependencies required at the runtime.
What are the best practices of Hyperapp?
This example is a counter that can be incremented or decremented -
import { h, app } from "hyperapp"
const state = {
count: 0
}
const actions = {
down: value => state => ({ count: state.count - value }),
up: value => state => ({ count: state.count + value })
}
const view = (state, actions) => (
<div>
<h1>{state.count}</h1>
<button onclick={() => actions.down(1)}>-</button>
<button onclick={() => actions.up(1)}>+</button>
</div>
)
app(state, actions, view, document.body)
Hyperapp consists of two-function APIs, i.e. hyperapp.h and hyperapp.app. hyperapp.h returns a new virtual DOM (Document Object Model) node tree and hyperapp.app mounts a new app in the specified DOM element. It's possible to use Hyperapp "headless" without a component, which can be helpful when implementing the unit test in the application. This example assumes that a JavaScript compiler like Babel or TypeScript and a module bundler like Parcel, Webpack, etc. are used. If JSX is used, the JSX transform plugin is installed and the pragma option is added to the .babelrc file.
{
"plugins": [["@babel/plugin-transform-react-jsx", { "pragma": "h" }]]
}
JSX is a language used to write HTML tags interspersed with JavaScript. Since browsers don't understand JSX, use a compiler to transform it into hyperapp.h function calls under the hood.
const view = (state, actions) =>
h("div", {}, [
h("h1", {}, state.count),
h("button", { onclick: () => actions.down(1) }, "-"),
h("button", { onclick: () => actions.up(1) }, "+")
])
Note that JSX preprocessor not required for building applications with HyperApp. One can use hyperapp.h directly and without a compilation step as shown above. Other alternatives to JSX include @hyperapp/HTML, hyperx, t7 and ijk.Hyperapp Tools
The tools required to get started with the HyperApp framework -Conclusion of working with Hyperapp
HyperApp web applications are interactive and responsive. They follow Powerpack's three principles, one-way data flow, JSX, and virtual DOM, to make the app more flexible and robust. The HyperApp framework is based on Elm architecture. Other Elm-based frameworks include React and Redux.
- Explore Design Patterns in TypeScript and JavaScript
- Read more about Kubernetes Native Serverless Framework.
Next Steps with Hyperapp Working
Talk to our experts about implementing compound AI system, How Industries and different departments use Agentic Workflows and Decision Intelligence to Become Decision Centric. Utilizes AI to automate and optimize IT support and operations, improving efficiency and responsiveness.